User-Based Licensing
User-based licensing is a contemporary approach to software or service provisioning, wherein access and usage rights are granted on a per-user basis.
Unlike traditional licensing models that focus on the number of devices or installations, user-based licensing centers around the actual individuals who will be utilizing the product or service.
This model has gained popularity due to its flexibility and scalability, providing businesses and organizations with a more efficient and cost-effective way to manage their software resources.
By the end of this tutorial, you should understand how to create a login feature by activating a license, how to create a logout feature by deactivating a license, and how to routinely perform online checks on your user-based license from your program.
Email and password of users, can be found on the licenses page. Utilizing a user-based license requires users to be created and assigned to the license.
This is done by entering the user-based license, switching to the users tab, and clicking “assign license user”.
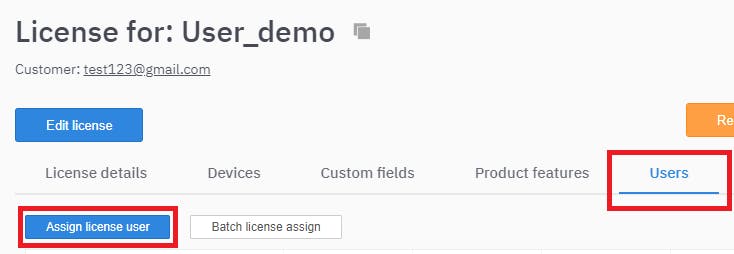
Configuration is a set of parameters or settings that are specific to a SDK and can include elements such as API keys,shared keys or product codes. More about Configuration here.
The local license is a copy of the license information that is saved on the end user's local computer. Users who have not activated the license on their end before will not have a local license. By default, the local license is stored: %USERPROFILE%\AppData\Local\LicenseSpring\”Product Code”\License.key.
You can read more about the local license file here.
The process of activating a license refers to binding a device to a license. On the LicenseSpring platform, activating the license increments the total activations counter within the license.
If a device already has a license, and you try to activate another license on that device, for the same product, that newly activated license will override the existing license, deleting it from the device. This will be on the developer to make sure that they check if a license exists on the device before a new activation:
Creating a login system with the user-based license is straightforward. You can ask the user to input their email and password combination. Once we have the user's credentials, we can then take that email/password combination and use it to activate our user-based license. How the user inputs their credentials is up to you.
It is recommended to perform a local license check at application open to confirm that the local license file belongs to the current device and has not been transferred. It is also useful to check whether the local license file has been tampered with and whether the local license is still valid. For more information on performing local license checks, see the Getting Started Tutorial.
User data such as email address, first name, last name, license ID, order ID, and order store ID can all be retrieved through the SDK. Initial passwords are also available on your local license file, but once the password has been changed, you will no longer be able to access that data using the LicenseSpring SDK. All this user data is stored and encrypted on your local license file. The SDK allows you to retrieve the data and decrypt it. For more on license encryption/decryption see our tutorial on Safety/Security Considerations.
Here is how to retrieve a "User Data" object that contains such information
We can allow users to change their password. This can be done by the License Manager through the platform, but we can also allow end-users to change their password using our SDK. To change the password, we will require the original/old password and a new, replacement password. If the old password is correct, and the new password is valid, then the user's password will be changed. Note: this method requires internet connection.
New accounts are created with a temporary, initial password. We can check if a license user is still using an initial password and prompt them to change their password using the following code:
To return the list of all the license users on every order by some customer use GetCustomerLicenseUsers method of the SDK. This method returns a list of LicenseUser objects that represents all license users associated with the customer.
Exception | Error code | Definition |
ConfigurationException | eInitializationError | Throws in case of something wrong with Configuration. E.g. wrong API or Shared key |
InvalidCredentialException | eInvalidCredential | This exception means that user or password is wrong |
LicenseActivationException | eActivationFailed | Rare exception, can arise if activation is limited to some device count or license transfer is prohibited |
LicenseNoAvailableActivationsException | eLicenseNoAvailableActivations | Throws when license has already been activated maximum number of times |
PasswordChangeNotAllowedException | ePasswordChangeNotAllowed | This exception can arise if password change is restricted by company policy |
Exception | Definition |
InvalidCredentialException | This exception means that user or password is wrong |
CustomerNotFoundException | In case customer does not exist |
InvalidAuthMethodException | Thrown when GetCustomerLicenseUsers method is called for key-based product |
PasswordChangeNotAllowedException | This exception can arise if password change is restricted by company policy |
MissingParametersException | If empty string is passed to ChangePassword method |

