Offline Licensing
In certain scenarios, some computers may lack an active internet connection or be behind a restrictive firewall, hindering online activation.
To address this, we have developed a mechanism that enables the activation and deactivation of licenses in an offline environment.
- Initialized LicenseManager (or LicenseHandler) with your configuration using the appropriate settings.
- Created a LicenseID using either LicenseID::fromKey or LicenseID::fromUser function, depending on the activation method you prefer.
Performing offline license activation using the LicenseSpring SDKs is a straightforward process. Follow the steps below to activate and deactivate licenses:
License Activation Process:
- Generate a request file by calling the createOfflineActivationFile function of LicenseManager. Provide the LicenseID you created earlier and specify the path where you want to save the request file. The function will return the path to the created file.
- Open the offline activation page and upload the request file you generated in the previous step. Once uploaded, download the offline activation file from this page.
- Activate the license by calling the activateLicenseOffline function of LicenseManager and passing the path to the offline activation file received from the website. The function will return a License object upon successful activation, or null if the activation process fails.
License Deactivation Process:
- Call the deactivateOffline function of the License object, and optionally provide the path where you want to save the deactivation request file. The function will return the path to the created file.
- Call the clearLocalStorage function of LicenseManager to delete the files created by the SDK to store license data.
- Open the offline activation page and upload the deactivation request file you generated in the previous step. Once uploaded, you will receive a message confirming that the license has been deactivated.
Let's get started!
To initiate offline license activation, the first step involves creating an offline activation request file.
You can accomplish this by utilizing the following function:
The provided function generates an offline activation request file for the specified key-based/user-based license, identified by LicenseID.
If you include a 'path' parameter, the file will be created with the specified path and name.
Note: If 'path' is not provided, the default location for the offline activation request file will be the desktop, and the default name will be "ls_activation.req": C:\Users%USERNAME%\Desktop\ls_activation.req.
Once you have generated your offline activation request file, the next step is to upload it to the LicenseSpring offline portal. It's essential to note that this process requires a computer with online access.
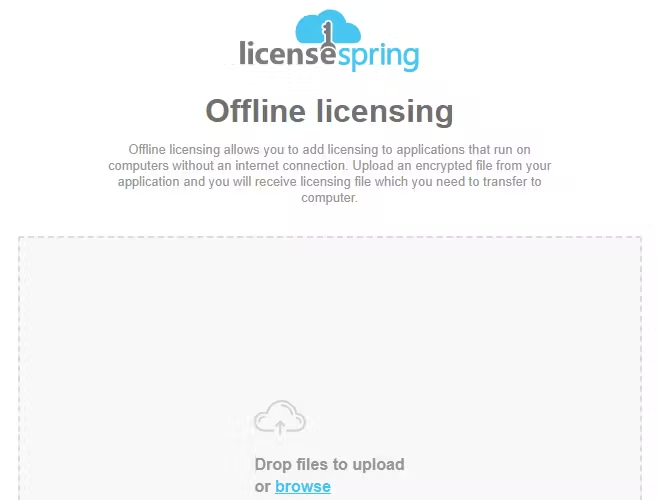
Upon uploading the request file, you will receive an offline activation response file named "ls_activation.lic." This response file will enable you to proceed with the offline activation process successfully.
To complete the offline activation process on a device, utilize the function activateLicenseOffline(path) as demonstrated below:
In the 'path' parameter, provide the name and path of the offline activation response file.
If 'path' is left empty, the function will search for the response file named "ls_activation.lic" on the desktop by default: C:\Users%USERNAME%\Desktop\ls_activation.lic.
By running this, you will have successfully activated the license offline on the device.
There is a checker for if the license is offline activated also, as shown below:
This Boolean checker returns true if offline activated, and false if not.
Guard files prevent users from using the same license request file and are automatically enabled within ExtendedOptions.
Guard files can be disabled in the ExtendedOptions constructor or by running the following method, where options is an ExtendedOptions object:
Once enabled, LicenseSpring generates a guard file in the same folder where the license and SDK logs are stored.
This guard file remains hidden and plays a crucial role in safeguarding the licensing process. Should anyone attempt to remove or alter this file, the license activation will fail as a protective measure.
The guard file is automatically created when you generate the request file for offline activation.
It must remain valid and present when a user submits the response file to ensure a successful activation process. The presence of the guard file is pivotal for maintaining the integrity and security of the offline activation procedure.
To determine the current status of the guard file, you can utilize the following method (where options is an ExtendedOptions object):
If a guard file is actively protecting the offline activation process guardFileTest returns true.
This method also exists within the Configuration object, so using the following (where config is a Configuration object) will yield an identical result:
Offline license refresh allows users to update their activated offline license, which is typically used when certain aspects of the license have been modified on the backend.
For instance, you might want to add a new feature without requiring license reactivation.
To perform an offline license refresh, locate the license on the LicenseSpring Platform and access the Devices tab.
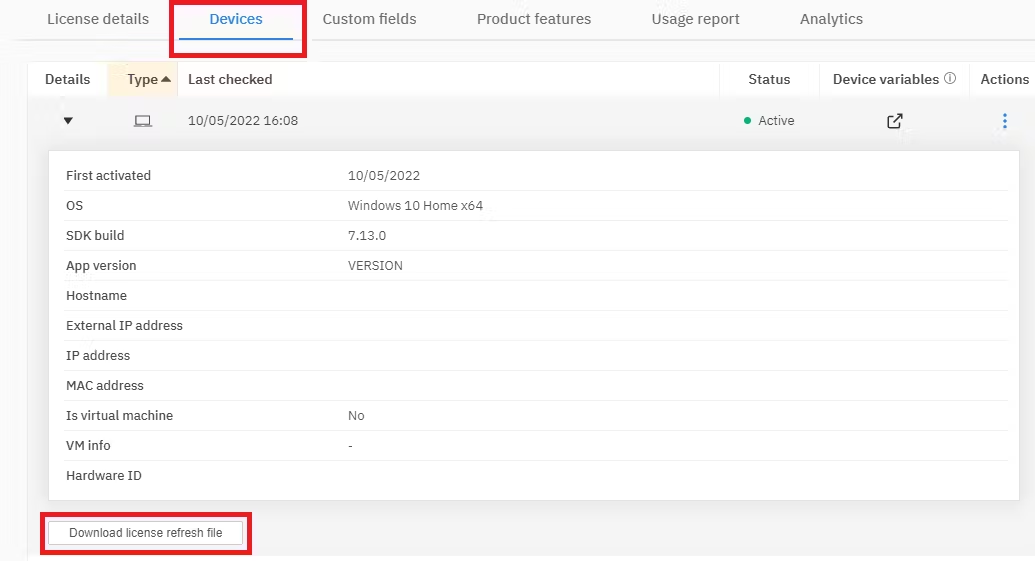
The line of code responsible for the update is as follows:
In the path parameter, you should provide the path to the license_refresh.lic file, which you downloaded from the page, as shown above.
updateOffline also has a secondary optional parameter, resetConsumpion, which is disabled by default. If this parameter is set to true, then this update also resets the consumption.
This method returns true if the license is successfully updated, and false otherwise.
Once a license has been activated, it can also be deactivated through a similar process. The function responsible for creating the offline deactivation request file is as follows:
Additionally, you have the option to provide an alternative path as an optional parameter to the deactivateOffline function, where the deactivation request file will be created and stored.
Note: If you leave this parameter empty, the default file path will be used, saving the file to the desktop.
To eliminate any local license data, after creating the deactivation file, we execute the following:
By performing these steps, you can efficiently deactivate the license and clear any associated local data.
First we load the local license, using getCurrentLicense whilst checking for any local license errors:
Similarly to creating a deactivation request file, we can select a path for this file to be created or use the default path by leaving the path as null in createOfflineActivationFile( licenseID, path ).
Note: If the path parameter is null, then the default path that createOfflineActivationFile( licenseID ) creates the file at is: 'C:\Users\%USERNAME%\Desktop\ls_deactivation.req'.
Now that we have submitted the offline activation file to the portal and downloaded the activation response file, we must activate our device locally.
After checking that our local license is not active, we run activateLicenseOffline( path ).
Note: If the path parameter is null, then the default path that activateLicenseOffline searches for the file is: 'C:\Users\%USERNAME%\Desktop\ls_deactivation.req'.
To verify the license locally on the device without connecting to the backend, we utilize the localCheck function in the following manner:
If license is a nullptr, it indicates that a local license does not exist. Therefore, it is crucial to first confirm the existence of a local license before proceeding with any operations related to the license.
The localCheck function also throws exceptions to indicate any encountered issues.
This feature proves beneficial in assessing the license's validity and detecting potential license tampering. If any irregularities are detected, the function will throw an exception.
To ensure license integrity, it is considered best practice to perform a localCheck (offline check) during each startup.
This practice helps confirm that the license file has not been copied from another computer and that the license is in a valid state.
A code sample that handles all potential exceptions is also provided below:
To update licenses offline, we use the updateOffline( path ) method as shown below, where license is a license object.
Just like the previous code sample, we can also first check whether license is a nullptr to confirm the local license exists before we attempt to update it.
We can also handle the specific exceptions thrown by updateOffline( path ) as shown below:
To deactivate a license offline, first ensure that the license is currently active. Then an offline deactivation request file will be created at the path defined in the parameter of deactivateOffline( path ).
Note: If the path parameter is null, then the default path that deactivateOffline() creates the file at is: 'C:\Users\%USERNAME%\Desktop\ls_deactivation.req'.
After creating the offline deactivation request file, it is vital that the local license file is deleted, using clearLocalStorage.
A full code sample is as follows:
Automating the offline activation process becomes achievable by utilizing the following API endpoints:
These endpoints provide developers with the necessary tools to seamlessly manage offline license activation, enabling users to access and activate licenses without relying on a constant internet connection.
Note: If you want to use these API endpoints directly, instead of using the SDK, please contact us for additional instructions.
Exceptions that might occur when performing offline license activation:
- SignatureMismatchException: Signature inside activation file is not valid.
- LocalLicenseException: Invalid activation file provided.
- ProductMismatchException: License product code does not correspond to configuration product code.
- DeviceNotLicensedException: License file does not belong to the current device.
The offline license activation process may encounter failure due to any of the following reasons:
- Total activations on the account have reached the maximum limit.
- The license is expired or disabled.
- Incorrect license credentials provided during activation.
- Incorrect product configuration, such as using a shared key, API key, or product code.
- The license is associated with an account using the free tier.
